Introduction
Generics were introduced in Java 1.5 with the purpose of identifying probable run time errors related to type at compile time itself. It provides stronger type checks at compile-time and makes a class, interface and methods type-safe. It also eliminates explicit type casting.
In the last post, we already learnt about Generics and Generic class in Java. In this post, we will learn –
- How to create a generic class with multiple type parameters?
- How to use both type parameters and concrete types in a Generic class?
- What is raw type of generic class?
Creating generic class with multiple parameters
We can use multiple type parameters in a generic class. Java convension says to single uppercase letter as type parameter. The most commonly used type parameter names are:
- E – Element (used extensively by the Java Collections Framework)
- K – Key
- N – Number
- T – Type
- V – Value
- S,U,V etc. – 2nd, 3rd, 4th types
Below class is an example of generic class with multiple type parameters and explicit parameters.
package genericsexamples; public class GenericClassWithMultipleParameters { private T s1; private S s2; private String s3; private int s4; public GenericClassWithMultipleParameters(T s1, S s2, String s3, int s4) { this.s1 = s1; this.s2 = s2; this.s3 = s3; this.s4 = s4; } public void printValues() { System.out.println(s1); System.out.println(s2); System.out.println(s3); System.out.println(s4); } }
We can use above class as below –
package genericsexamples; public class StoreValue { public static void main(String[] args) { GenericClassWithMultipleParameters newObj = new GenericClassWithMultipleParameters( "Amod", 10, "Mahajan", 1); newObj.printValues(); } }
Raw Type of generic class
If we instantiate a generic class without passing type arguments then the compiler will give you a warning stating that the generic class is a raw type. It will not give any error and stop you from using this class but again we will be stuck in the problems discussed in this post.
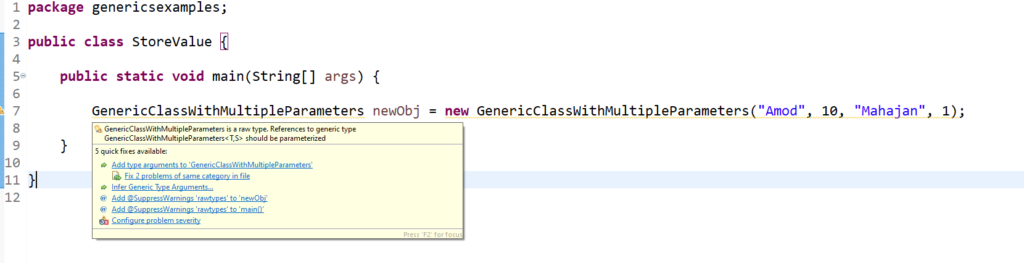
Type Parameters vs Type Arguments
When we create a generic class then we pass a type parameter but when we instantiate that generic class then we pass type arguments.
package genericsexamples; // T is generic parameter public class StoreAnyValueWithGenerics { private T value; public StoreAnyValueWithGenerics(T value) { this.value = value; } public T getValue() { return value; } }
//Integer is a type argument here StoreAnyValueWithGenerics s1 = new StoreAnyValueWithGenerics<>(10);
That’s all in this post. We are going to learn a lot about generics in upcoming posts.
If you have any doubt, feel free to comment below.
If you like my posts, please like, comment, share and subscribe to my YouTube channel.
#ThanksForReading
#HappyLearning